Advanced Git Aliases for Speed and Productivity
Discover how to create powerful Git aliases to streamline your workflow and boost productivity.
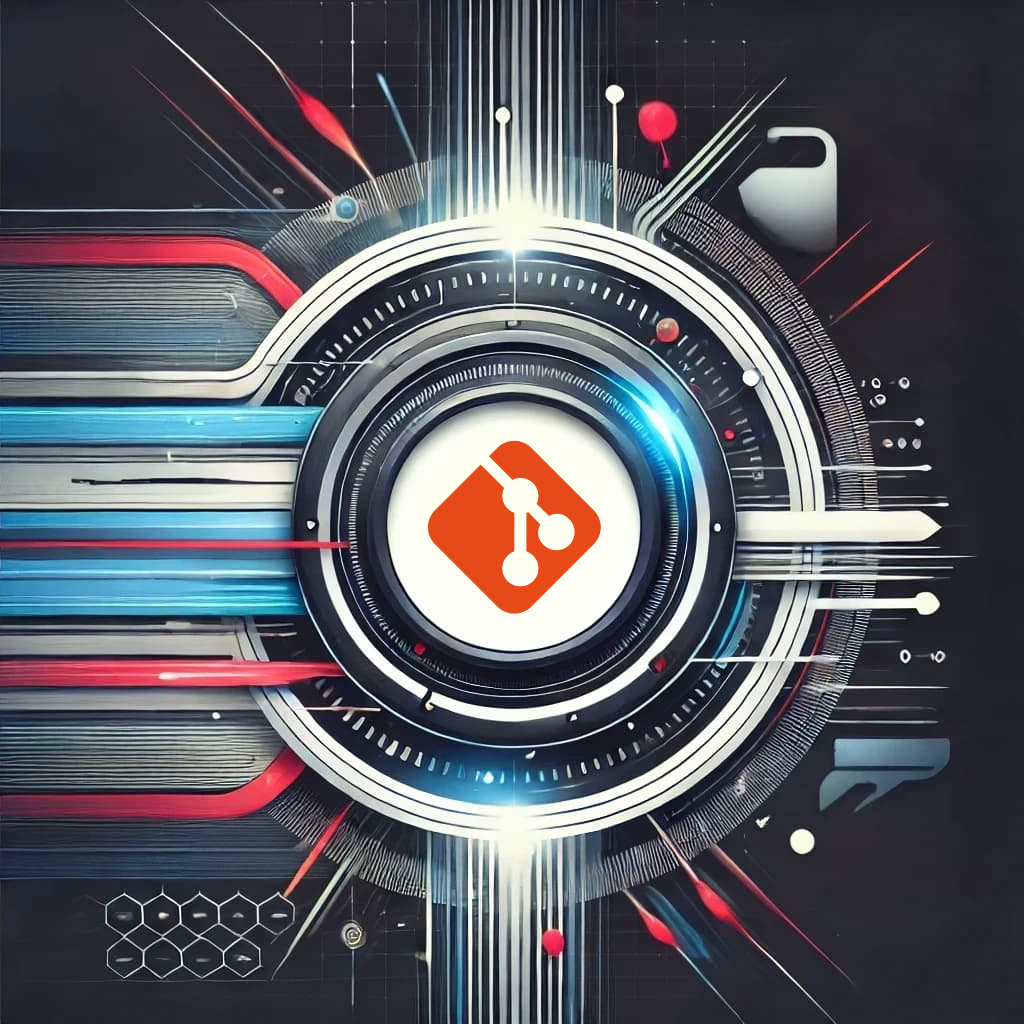
Git aliases are a powerful tool that can significantly enhance your productivity and streamline your workflow. In the previous article, the basics of Git aliases are covered and it's explained how to create simple shortcuts for common Git commands. In this article, advanced Git aliases that leverage shell scripting and custom commands to automate complex tasks and improve your efficiency will be explored.
How to run shell scripts in Git aliases
One of the most powerful features of Git aliases is the ability to execute shell scripts and custom commands directly from the command line. By combining Git commands with shell scripting, you can create sophisticated aliases that automate repetitive tasks and save you time and effort.
To run a shell script in a Git alias, you can use the !
operator followed by the shell command you want to execute. For example, to create an alias that displays the current branch and its status, you can define it like this:
git config --global alias.statusline "!git status && git branch"
When you run git statusline
, it will execute both git status
and git branch
commands sequentially, providing you with the information you need in a single output.
Alternatively, you can create a shell script file with your custom commands and execute it from the alias. Create a new directory for your scripts, for example, ~/scripts
, and save your shell scripts there.
For example, you can create a script named my-script.sh
in the ~/scripts
directory with the following content:
#!/bin/bash
echo "Hello, this is my custom script!"
Then, you can define an alias that runs this script like this:
git config --global alias.custom "!sh ~/scripts/my-script.sh"
When you run git custom
, it will execute the my-script.sh
script and display the output in your terminal.
Advanced Git aliases for speed and productivity
Now that you know how to run shell scripts in Git aliases, let's explore some advanced aliases that can help you work faster and more efficiently.
If you're using another platform or programming language for the script, you have to use the -c
option for the !sh
command to execute the string which comes after it. We will use this option in the following examples.
I decided to use Node.js for the following examples, but you can use any scripting language you prefer. To run the scripts, you need to have Node.js installed on your machine. Please download it from here nodejs.org.
Git Recent Branch Names
If you frequently switch between branches in your Git repository, it can be helpful to have a quick overview of the most recently used branch names. You can create an alias that lists the recent branch names ("rb") you checked out like this:
git config --global alias.rb "!sh -c 'node ~/scripts/recent_branches.js'"
In this example, we are executing a Node.js script named recent_branches.js
that retrieves and displays the recent branch names. You can customize the script to fit your needs and display additional information if desired.
You can find the script for this alias in the GitHub Gist.
The result will look like this:
Git alias for recent branch names
Git Calendar
Imagine you're new to a project and want to get some history about the contributors and the commits in the past. How long is the project already developed? Was there a time where a lot of commits were made? You can create a calendar view of the commits with the following alias:
git config --global alias.rb "!sh -c 'node ~/scripts/calendar.js "$@"' -"
The script for this alias is available in the GitHub Gist.
The result looks like this:
Git alias for calendar view of commits
For every commit which has been made in the repository, a colored square is displayed. Here you can see the history of the project at a glance. It's quite similar to the GitHub contribution graph, but you can use it locally, and it's more focused on single repositories than on the whole GitHub account with all of its contributions.
At the end of the alias, you can find $@' -
. This part is used to pass the parameters to the script. These parameters are used to filter the commits by year or author. For example, you can filter the commits by year and author like this:
git cal --year 2018 --author "Philipp Kief"
The result will look like this:
Git alias for calendar view of commits with author filter
Git Backmerge
A backmerge is a merge that brings changes from the main branch into the feature branch. This should be done regularly to keep the feature branch up to date with the latest changes from the main branch. Sometimes the branch structure can be nested and it's not easy to find out which branch should be merged into which. To simplify this process, you can create an alias that performs a backmerge (short "bm") for you:
git config --global alias.bm "!sh -c 'node ~/scripts/backmerge.js "$@"' -"
The script for this alias can be found in the GitHub Gist.
You can run it like this:
git bm
If the script will be executed for the first time in a repository, it will ask you for the main branch name:
Git alias for backmerge
After that, the script wants to confirm if the branch should be merged into the branch you're currently on:
Git confirm backmerge
If the backmerge was successful, you will see the following output: ✅ Backmerge from "dev" into "feat/accessibility" successful
.
From now on the script wont ask again for the main branch name. You can simply run git bm
and press enter to perform the backmerge.
To change the default branch for the backmerge, you can run the alias with the parameter --set-default-branch
:
git bm --set-default-branch main
The result will look like this:
Changing the default branch
Additionally, you can read the manual for the alias with the parameter --manual
:
git bm --manual
Here you can read all information about the alias and its parameters.
Git checkout default branch
Based on the backmerge alias, you can create an alias that checks out the default branch. This can be helpful if you want to switch back to the main branch quickly:
git config --global alias.default "!git checkout $(git config --get backmerge.defaultBranch)"
With this alias, you can run git default
to switch to the default branch.
Conclusion
Using scripts can give you much more flexibility and power when creating Git aliases. By combining Git commands with shell scripting, you can automate complex tasks, customize the output, and create powerful shortcuts that fit your workflow. This article demonstrated how to do so with a few helpful examples, but the possibilities are endless. Experiment with different scripts and commands to create advanced Git aliases that boost your productivity and make your work more efficient.